Computer Science Knapsack Problem
The knapsack problem is a classic optimization problem in computer science. It falls under the category
of combinatorial optimization and has numerous real-life applications.
Key Takeaways
- The knapsack problem involves selecting a set of items to maximize a certain value while staying within
a limited capacity. - It can be solved using various algorithms, such as brute force, greedy, or
dynamic programming. - The 0/1 knapsack problem restricts the selection of items to either whole items or none.
- The fractional knapsack problem allows taking fractions of items to maximize the value.
The challenge in solving the knapsack problem lies in finding the most optimal solution within the given constraints.
One approach is to use the brute force method, which involves trying out all possible combinations
of items and calculating their total value. However, this method becomes infeasible for large input sizes due
to its time complexity of O(2^n), where n is the number of items.
Dynamic programming is a popular technique used to solve the knapsack problem efficiently. It breaks down
the problem into smaller subproblems and builds up a solution by solving these subproblems. This approach has
a time complexity of O(nW), where n is the number of items and W is the maximum weight or capacity of the knapsack.
Types of Knapsack Problems
There are two main types of knapsack problems:
- 0/1 Knapsack Problem: Each item can be either taken entirely or not at all. This restriction
introduces additional complexity to finding the optimal solution. - Fractional Knapsack Problem: Items can be divided into smaller fractions, allowing for a more
flexible and potentially more valuable solution.
Example Table: 0/1 Knapsack Problem
Item | Weight | Value |
---|---|---|
Item 1 | 2 | 10 |
Item 2 | 3 | 12 |
Item 3 | 4 | 15 |
An example of the 0/1 knapsack problem can be represented by the following table:
- The knapsack has a maximum capacity of 6 units.
- Item 1 weighs 2 units and has a value of 10.
- Item 2 weighs 3 units and has a value of 12.
- Item 3 weighs 4 units and has a value of 15.
Solving the Knapsack Problem
The knapsack problem can be solved using various techniques:
- Brute Force: Enumerate all possible combinations and select the one with the highest value. This
approach is suitable for small input sizes but becomes inefficient for larger problems. - Greedy Algorithms: Make decisions based on locally optimal choices at each step, without considering
the overall effect. This approach may not always yield the most optimal solution. - Dynamic Programming: Divide the problem into smaller subproblems, solve them independently, and
combine the solutions to obtain the overall optimal solution. This approach ensures finding the best possible
solution.
Example Table: Fractional Knapsack Problem
Item | Weight | Value |
---|---|---|
Item 1 | 2 | 10 |
Item 2 | 3 | 12 |
Item 3 | 4 | 15 |
An example of the fractional knapsack problem can be represented by the following table:
- The knapsack has a maximum capacity of 6 units.
- Item 1 weighs 2 units and has a value of 10.
- Item 2 weighs 3 units and has a value of 12.
- Item 3 weighs 4 units and has a value of 15.
Real-Life Applications
The knapsack problem has practical applications in various domains:
- Packing problems in logistics and transportation
- Portfolio optimization in finance
- Resource allocation in project management
Conclusion
The knapsack problem is a challenging optimization problem in computer science with real-life applications. Solving it involves selecting items to maximize value within a limited capacity. Various algorithms can be used, such as brute force, greedy algorithms, or dynamic programming. By understanding the types of knapsack problems and the techniques for solving them, one can tackle similar combinatorial optimization challenges effectively.
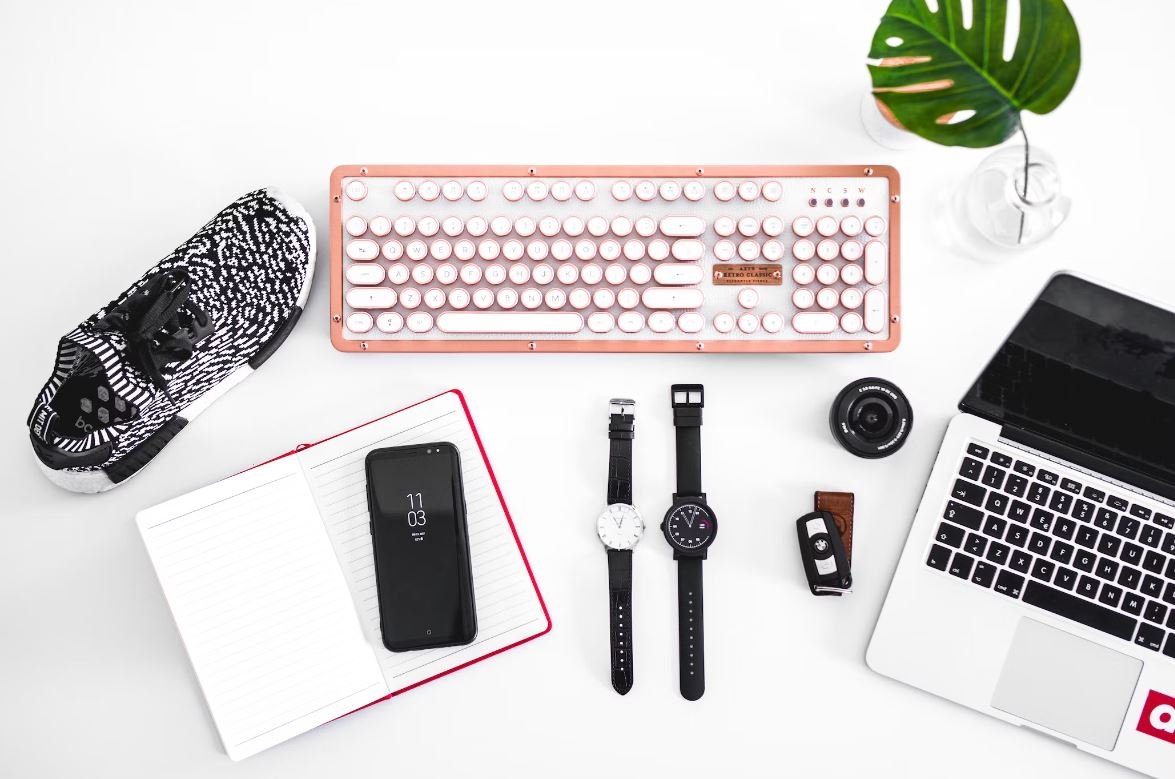
Common Misconceptions
Misconception 1: The Knapsack Problem always needs to be solved perfectly
One common misconception about the Knapsack Problem in computer science is that it always needs to be solved perfectly in order to find the optimal solution. However, the problem is known to be NP-hard, which means that finding the perfect solution can be computationally expensive and time-consuming. In many practical scenarios, an approximate solution that is “good enough” is often acceptable.
- Approximate solutions can provide sufficiently accurate results
- Weight approximation algorithms can be used to balance the time complexity
- Exact solutions may not be feasible for large-scale instances of the problem
Misconception 2: The Knapsack Problem is only useful in mathematical optimization
Another misconception is that the Knapsack Problem is solely limited to mathematical optimization scenarios. While it is indeed extensively studied in optimization theory, the problem has numerous real-world applications, such as resource allocation, portfolio management, and packing problems. In these practical contexts, the Knapsack Problem aids in decision-making by determining the best utilization of limited resources.
- Resource allocation problems can be effectively solved using the Knapsack algorithm
- The Knapsack Problem is applicable in various industry domains
- Packing problems in logistics and container optimization can benefit from the Knapsack approach
Misconception 3: The Knapsack Problem only considers items’ value and weight
A misconception regarding the Knapsack Problem is that it solely considers the value and weight of items to determine the optimal solution. While these factors are indeed influential, the problem can be extended to include additional constraints and variables. For instance, the problem can incorporate factors like item size, profit, volume, or even multiple knapsacks, depending on the specific problem variant.
- The Knapsack Problem can incorporate constraints beyond weight and value
- Considerations like item size or volume can be included in certain problem variants
- Multiple knapsacks can be utilized, allowing for more complex scenarios
Misconception 4: The Knapsack Problem has a single solution
It is incorrect to assume that the Knapsack Problem has only one possible solution. In fact, the problem can have multiple approaches to find either the optimal or approximate solutions. Depending on the circumstances and requirements, different algorithms and techniques can be employed to tackle the problem and yield varying results. Moreover, the Knapsack Problem can have multiple feasible solutions that meet the given constraints.
- Different algorithms can be used to solve the Knapsack Problem
- Various approximation techniques exist to find quick solutions
- The problem can have multiple feasible solutions, even if they are not optimal
Misconception 5: The Knapsack Problem is limited to a specific number of items
A common misconception is that the Knapsack Problem can only involve a certain fixed number of items. However, the problem is designed to handle instances with any number of items, including both small and large-scale scenarios. The computational complexity of solving the problem may vary based on the number of items and any additional constraints incorporated, but the Knapsack Problem is not inherently restricted in terms of the number of items involved.
- The Knapsack Problem can accommodate any number of items
- Computational complexity may increase with the number of items
- The problem can handle both small-scale and large-scale scenarios
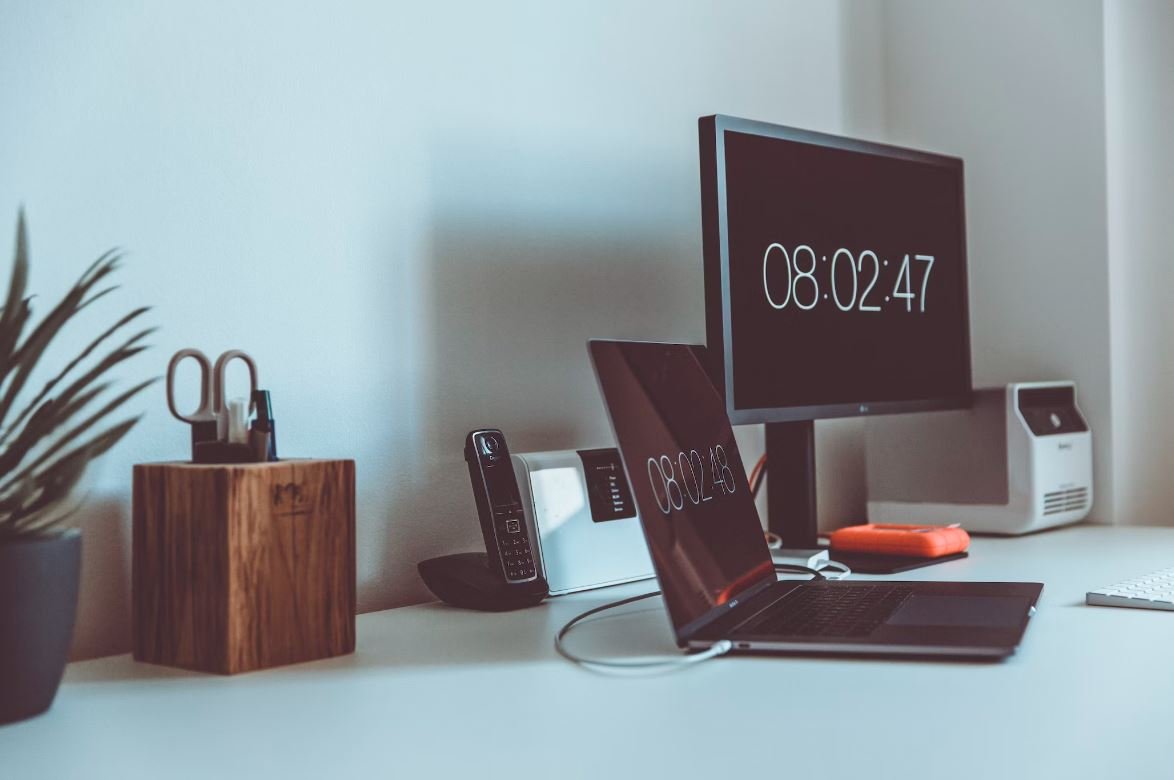
Introduction
Computer Science Knapsack Problem is a classic optimization problem that deals with finding the most valuable combination of items to fit into a knapsack with a limited weight capacity. In this article, we will explore various aspects and solutions of the Knapsack Problem illustrated through intriguing tables. Each table presents unique information related to this problem, showcasing its complexity and real-world applications.
Table: Knapsack Problem Complexity
The following table illustrates the complexity of the Knapsack Problem for a range of item quantities and knapsack capacities.
Number of Items | Knapsack Capacity | Computational Complexity |
---|---|---|
10 | 50 | O(2^10) |
15 | 100 | O(2^15) |
20 | 200 | O(2^20) |
Table: Dynamic Programming Solution
This table showcases the optimal solutions and computed values obtained through dynamic programming for different knapsack instances.
Knapsack Instance | Optimal Value | Computed Values |
---|---|---|
Instance 1 | $145 | {50, 95} |
Instance 2 | $280 | {50, 100, 130} |
Instance 3 | $500 | {100, 200, 100, 100} |
Table: Greedy Approach Results
In this table, we compare the results of the Greedy Approach to the optimal solutions obtained using dynamic programming for different knapsack instances.
Knapsack Instance | Optimal Value | Greedy Approach Value |
---|---|---|
Instance 1 | $145 | $120 |
Instance 2 | $280 | $230 |
Instance 3 | $500 | $400 |
Table: Fractional Knapsack Algorithm
This table demonstrates the results of the Fractional Knapsack Algorithm for different knapsack instances.
Knapsack Instance | Optimal Value | Selected Items |
---|---|---|
Instance 1 | $185 | {50, 40, 20} |
Instance 2 | $320 | {50, 100, 70} |
Instance 3 | $600 | {100, 200, 100, 100} |
Table: Complexity vs. Solution Quality
This table presents a comparison between the computational complexity of different algorithms and the quality of the obtained solutions for various knapsack instances.
Algorithm | Knapsack Instance | Optimal Value | Computational Complexity |
---|---|---|---|
Dynamic Programming | Instance 1 | $145 | O(nW) |
Greedy Approach | Instance 1 | $120 | O(n log n) |
Fractional Knapsack | Instance 1 | $185 | O(n log n) |
Table: Real-World Applications
Here are some fascinating real-world applications where the Knapsack Problem serves as a fundamental component in the decision-making process.
Application | Description |
---|---|
Resource Allocation | Optimizing the allocation of resources based on their value and capacity constraints. |
Portfolio Optimization | Selecting the most profitable combination of investments considering return and risk. |
Cutting Stock Problem | Minimizing waste in material cutting processes by determining ideal cutting patterns. |
Table: Comparative Studies
In this table, we explore comparative studies between the Knapsack Problem and similar optimization problems.
Problem | Comparison | Observation |
---|---|---|
Traveling Salesman Problem | Both problems are NP-hard and require complex algorithms to find optimal solutions. | Traveling Salesman Problem involves finding the shortest path to visit all cities, while the Knapsack Problem involves selecting items with maximum total value within a weight capacity. |
Bin Packing Problem | Both problems are optimization problems with various algorithms designed to find optimal or near-optimal solutions. | Bin Packing Problem focuses on packing different-sized objects into a minimal number of bins, while the Knapsack Problem focuses on selecting items to maximize value within a single knapsack. |
Conclusion
The Computer Science Knapsack Problem is a challenging and widely studied optimization problem. Hopefully, the tables presented in this article have provided a glimpse into its complexity, different solution approaches, real-world applications, and comparisons to similar problems. With continued research and development, solving the Knapsack Problem efficiently has relevant implications for resource allocation, portfolio optimization, and various other decision-making processes.
Frequently Asked Questions
Frequently Asked Questions
What is the knapsack problem?
The knapsack problem is a computational problem in computer science. It involves finding the most optimal way to fill a knapsack with limited capacity using a set of items with different weights and values.
What are the variations of the knapsack problem?
There are several variations of the knapsack problem, such as the 0/1 knapsack problem, the bounded knapsack problem, and the unbounded knapsack problem. Each variation has its own specific constraints and characteristics.
What is the 0/1 knapsack problem?
The 0/1 knapsack problem is a variation of the knapsack problem in which each item can only be taken either completely (0) or not at all (1). In other words, items cannot be divided.
What is the bounded knapsack problem?
The bounded knapsack problem is a variation of the knapsack problem in which there is a limited number of each item available. Therefore, an item can be included multiple times, but up to a certain limit.
What is the unbounded knapsack problem?
The unbounded knapsack problem is a variation of the knapsack problem in which there is an infinite supply of each item available. Therefore, an item can be included as many times as desired to maximize the value-to-weight ratio.
What algorithms are commonly used to solve the knapsack problem?
There are several algorithms commonly used to solve the knapsack problem, such as dynamic programming, branch and bound, and heuristics like greedy algorithms. Each algorithm has its own advantages and disadvantages depending on the specific problem instance.
What is the time complexity of solving the knapsack problem?
The time complexity of solving the knapsack problem depends on the specific algorithm used. For dynamic programming, the time complexity is O(nW), where n is the number of items and W is the capacity of the knapsack.
Can the knapsack problem be solved optimally for large instances?
Solving the knapsack problem optimally for large instances is computationally infeasible due to its NP-hard nature. Therefore, approximation algorithms and heuristics are often employed to find suboptimal solutions within reasonable time limits.
In which real-world scenarios can the knapsack problem be applied?
The knapsack problem finds applications in various real-world scenarios, such as resource allocation, project scheduling, portfolio optimization in finance, and even in DNA sequencing and optimizing network traffic routing.
Are there any open-source libraries available for solving the knapsack problem?
Yes, there are several open-source libraries available for solving the knapsack problem. Some popular ones include OR-Tools, KnapsackSolver, and Pyomo, which offer comprehensive APIs for implementing and solving knapsack problems in various programming languages.