Computer Science XI Syllabus
Computer Science XI is an essential course for students interested in the field of computer science. It covers a wide range of topics and provides a strong foundation for further studies in the field. This article provides an overview of the Computer Science XI syllabus and highlights key concepts and skills covered in the course.
Key Takeaways
- Computer Science XI covers a wide range of topics including programming languages, data structures, algorithms, and computer networks.
- The course focuses on problem-solving skills and algorithmic thinking.
- Students will have hands-on experience with programming languages such as Java and Python.
- The syllabus is designed to provide a strong foundation for further studies in computer science.
1. Introduction to Computer Science: This section provides an overview of computer science as a field of study and its importance in today’s world. *Understanding the impact of technology on society is vital in the digital age.*
2. Programming Languages: Students will learn and practice programming in languages like *Java and Python*. They will understand the concepts of variables, data types, control structures, and functions.
3. Data Structures: This topic introduces students to various data structures such as arrays, linked lists, stacks, queues, and trees. *Data structures allow efficient storage and manipulation of data.*
4. Algorithms: Students will study different algorithms, including searching, sorting, and graph algorithms. *Algorithms are the backbone of computer science, solving complex problems efficiently.*
Tables:
Language | Usage |
---|---|
Java | Widely used in enterprise applications and Android development |
Python | Popular for web development, data analysis, and artificial intelligence |
Data Structure | Description |
---|---|
Arrays | Stores a fixed-size sequential collection of elements of the same type |
Linked Lists | Consists of nodes where each node contains a data field and a reference to the next node |
Stacks | Follows the Last-In-First-Out (LIFO) principle |
Algorithm | Description |
---|---|
Binary Search | Finds the position of a target value within a sorted array |
Bubble Sort | Sorts a sequence of elements in ascending or descending order |
Depth-First Search | Traverses or searches a graph/tree recursively |
5. Computer Networks: This section covers the fundamentals of computer networks, including the OSI model, TCP/IP protocols, network topologies, and network security. *Understanding how data is transmitted over networks is crucial in today’s interconnected world.*
6. Databases: Students will learn the basics of database management systems, SQL queries, and database design principles. *Databases are essential for storing and managing large amounts of structured data.*
7. Web Development: This topic introduces students to web technologies such as HTML, CSS, and JavaScript. They will learn how to create interactive and dynamic web pages. *Web development skills are in high demand in the job market.*
Conclusion:
Computer Science XI provides a comprehensive understanding of computer science principles and practical skills. From programming languages to data structures and algorithms, the course equips students with a strong foundation for further studies or a career in the field. Whether you’re interested in software development, data analysis, or cybersecurity, Computer Science XI lays the groundwork for success in the ever-evolving world of technology.
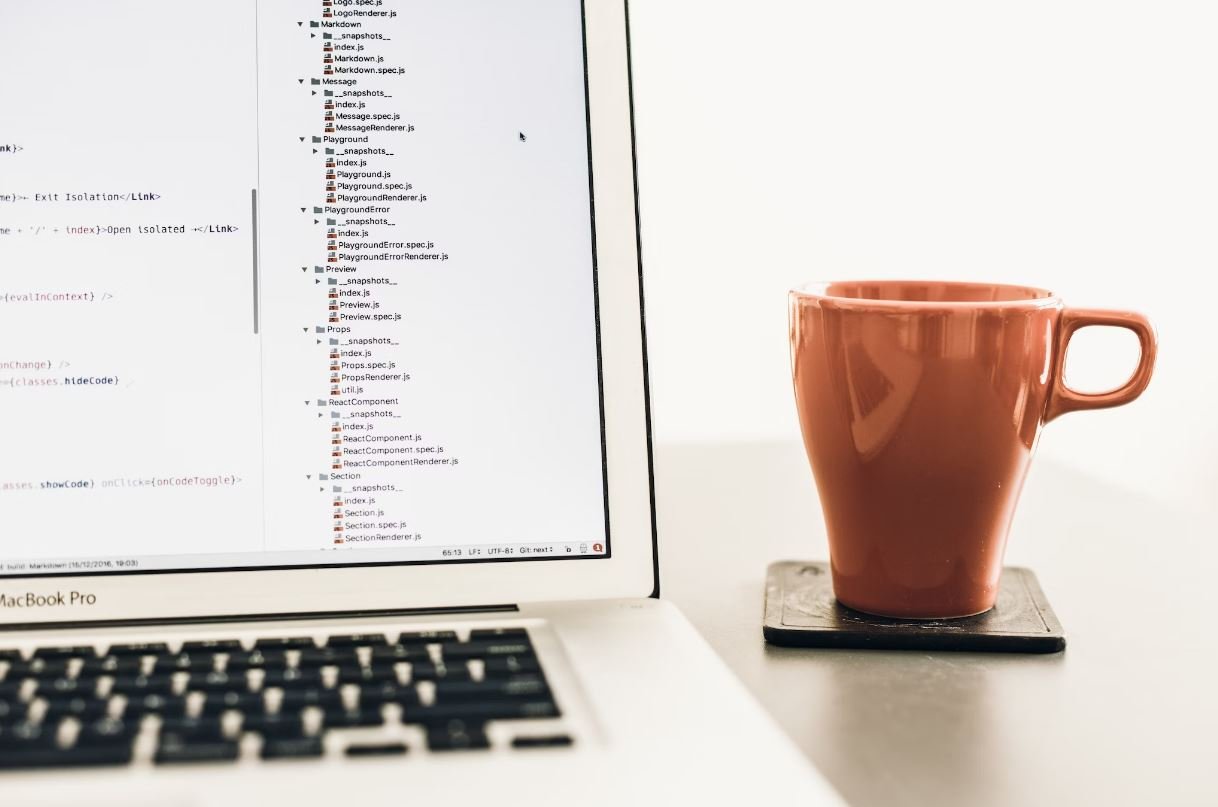
Common Misconceptions
There are several common misconceptions that people have about the Computer Science XI Syllabus. Let’s address some of these misconceptions and clear up any confusion.
Misconception 1: Computer Science is all about coding
– Computer Science is a broad and multidisciplinary field that encompasses much more than just coding.
– The syllabus includes topics such as algorithms, computer architecture, data structures, and networking, among others.
– Coding is an important aspect of computer science, but it is only one component of the entire subject.
Misconception 2: Computer Science requires advanced math skills
– While some areas of computer science involve complex mathematical concepts, not every aspect of the subject requires advanced math skills.
– The syllabus covers fundamental math concepts such as logic, sets, and functions, which are essential for understanding computer science principles.
– Many computer science concepts can be understood and implemented without deep mathematical knowledge.
Misconception 3: Computer Science is only for tech-savvy individuals
– Computer Science is not limited to individuals who are already tech-savvy.
– The syllabus is designed to introduce concepts and build skills from the ground up, making it accessible to beginners.
– With dedication and practice, anyone can develop their computer science skills regardless of their initial level of technical knowledge.
Misconception 4: Computer Science is a solitary pursuit
– Although computer science projects sometimes require focused individual work, collaboration and teamwork are highly valued and emphasized.
– The syllabus includes opportunities for group work, code reviews, and discussions, fostering a collaborative learning environment.
– Computer science professionals often work in teams to tackle complex problems and create innovative solutions.
Misconception 5: Computer Science is only useful for a career in software development
– Computer Science skills are valuable in a wide range of fields, not just software development.
– The syllabus covers concepts that are applicable in areas such as data analysis, cybersecurity, artificial intelligence, and many more.
– Computer Science knowledge can enhance problem-solving and critical-thinking abilities, which are transferable skills for various industries.
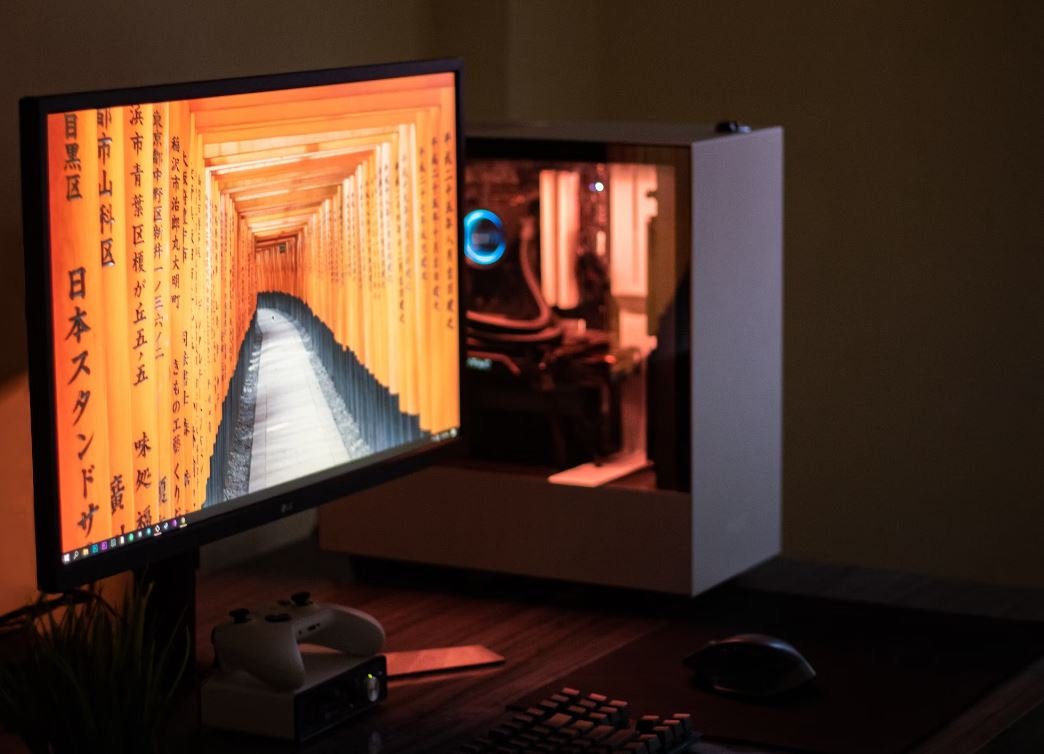
Syllabus Overview
The Computer Science XI syllabus covers various topics in computer science, including programming, data structures, algorithms, and computer networks. The following tables provide detailed information about the different components of the syllabus.
Programming Languages
The table below showcases popular programming languages that students will learn during the course:
Language | Popularity | Usage |
---|---|---|
C++ | High | General-purpose, widely used for game development and system programming. |
Java | Very High | Used for building Android apps, enterprise software, and large-scale web applications. |
Python | Extremely High | Known for its simplicity and readability, suitable for web development, data analysis, and artificial intelligence. |
JavaScript | Very High | The language of the web, used for front-end and back-end web development. |
Data Structures
The syllabus includes important data structures that lay the foundation for efficient algorithm design:
Data Structure | Definition | Example |
---|---|---|
Array | A collection of elements stored at contiguous memory locations. | [1, 2, 3, 4, 5] |
Linked List | A linear data structure where elements are connected via links. | 1 -> 2 -> 3 -> 4 -> 5 |
Stack | A data structure based on the Last-In-First-Out (LIFO) principle. | [5, 4, 3, 2, 1] |
Queue | A data structure based on the First-In-First-Out (FIFO) principle. | [1, 2, 3, 4, 5] |
Algorithm Complexity
This table presents the time complexity of common algorithms, helping students understand the efficiency of different algorithms:
Algorithm | Time Complexity | Explanation |
---|---|---|
Bubble Sort | O(n^2) | A simple sorting algorithm with a quadratic time complexity. |
Quick Sort | O(n log n) | An efficient divide-and-conquer sorting algorithm. |
Dijkstra’s Algorithm | O(V^2) | A graph traversal algorithm used to find the shortest path. |
Binary Search | O(log n) | An efficient search algorithm for sorted arrays. |
Computer Networks
The syllabus covers the fundamental concepts of computer networks and introduces protocols and technologies:
Concept | Description | Example |
---|---|---|
IP Addressing | A unique identifier assigned to each device connected to a network. | 192.168.0.1 |
Routing | The process of selecting a path for network traffic. | Routing table |
HTTP Protocol | A protocol used for transmitting web pages and other resources. | http://www.example.com |
DNS | The system translating domain names into IP addresses. | www.google.com -> 172.217.168.206 |
Boolean Logic
The syllabus introduces the principles of Boolean logic, necessary for understanding digital circuits:
Gate | Truth Table | Description |
---|---|---|
AND | 0 0 -> 0 0 1 -> 0 1 0 -> 0 1 1 -> 1 |
Output is true if and only if all inputs are true. |
OR | 0 0 -> 0 0 1 -> 1 1 0 -> 1 1 1 -> 1 |
Output is true if at least one input is true. |
NOT | 0 -> 1 1 -> 0 |
Output is the inverse of the input. |
XOR | 0 0 -> 0 0 1 -> 1 1 0 -> 1 1 1 -> 0 |
Output is true if an odd number of inputs are true. |
Software Development Life Cycle (SDLC)
The following table outlines the different phases of the SDLC:
Phase | Activities |
---|---|
Requirements Gathering | Identifying and documenting software requirements. |
Design | Creating software architecture and detailed design specifications. |
Coding | Implementing the software based on the design specifications. |
Testing | Verifying and validating the software for quality and functionality. |
Operating Systems
The syllabus includes an introduction to different operating systems and their features:
Operating System | Main Features |
---|---|
Windows | Graphical user interface, multitasking, virtual memory. |
Linux | Open-source, stability, security. |
macOS | Sleek design, compatibility with Apple hardware, Unix-based. |
Android | Mobile operating system, wide app compatibility. |
Artificial Intelligence
The syllabus explores the fascinating field of artificial intelligence and its applications:
Application | Domain | Example |
---|---|---|
Speech Recognition | Natural Language Processing | Virtual assistants like Siri, Alexa, and Google Assistant. |
Image Classification | Computer Vision | Automatic categorization of images based on their content. |
Autonomous Vehicles | Robotics | Self-driving cars and drones. |
Recommendation Systems | Machine Learning | Personalized movie or product recommendations on online platforms. |
Conclusion
The Computer Science XI syllabus encompasses a wide range of topics, from programming languages and data structures to computer networks and artificial intelligence. By studying these elements, students will gain a solid foundation in computer science, enabling them to pursue diverse career paths in the technology industry. The syllabus provides a comprehensive and exciting journey into the world of computing, fostering critical thinking, problem-solving, and creativity in students. Mastery of these concepts will equip students with the necessary skills to tackle real-world challenges in the digital age.
Frequently Asked Questions
How can I access the Computer Science XI Syllabus?
You can access the Computer Science XI Syllabus by visiting your academic institution’s website or contacting your school’s administration department.
What are the main topics covered in the Computer Science XI Syllabus?
The main topics covered in the Computer Science XI Syllabus typically include programming concepts, algorithms, data structures, software engineering, computer architecture, operating systems, and networking.
Are there any prerequisites for studying Computer Science XI?
While prerequisites can vary based on educational institutes, it is generally recommended to have a basic understanding of mathematics and logical reasoning skills before studying Computer Science XI.
What programming languages are taught in Computer Science XI?
Common programming languages taught in Computer Science XI include Python, Java, C++, and HTML/CSS. The specific choice of programming languages may vary depending on the curriculum followed by your institution.
What resources or textbooks are recommended for Computer Science XI?
It is advisable to consult with your academic institution or course instructor for the recommended resources or textbooks for Computer Science XI. Some popular textbooks include “Introduction to Java Programming” by Y. Daniel Liang, “Python Crash Course” by Eric Matthes, and “Data Structures and Algorithms in Java” by Robert Lafore.
What assessments can I expect in Computer Science XI?
Assessments in Computer Science XI may include written exams, practical programming assignments, projects, and group presentations. The weightage and specific format of assessments can vary depending on your educational institution and curriculum.
Can I pursue a career in Computer Science after studying Computer Science XI?
Yes, studying Computer Science XI can be a stepping stone towards pursuing a career in the field of computer science. It provides a solid foundation in programming, algorithms, and other fundamental concepts, which are highly relevant in various computer science professions.
What are the career opportunities after studying Computer Science XI?
Completing Computer Science XI opens up a wide range of career opportunities. Some popular career options include software developer, web developer, data analyst, system analyst, database administrator, and network engineer, among others.
Is it necessary to have prior programming experience for Computer Science XI?
No, having prior programming experience is not a prerequisite for Computer Science XI. The course is designed to cater to students with varying levels of programming knowledge. However, having an interest in logical reasoning and problem-solving can greatly benefit your learning journey.
Can I continue studying Computer Science at a higher level after completing Computer Science XI?
Absolutely! After completing Computer Science XI, you can pursue higher education in the field of computer science or related disciplines. This can include undergraduate degrees in computer science, software engineering, or pursuing a specialization or master’s degree in a specific area of interest within the field.