Language Processing in Compiler Design
Compiler design involves converting high-level programming languages into machine code that can be executed by a computer. Central to this process is language processing, which involves analyzing and understanding the source code to generate an executable output.
Key Takeaways
- Language processing is a crucial aspect of compiler design.
- It involves analyzing and understanding the source code.
- The output generated is machine code that can be executed by a computer.
Language processing in compiler design encompasses several stages, including lexical analysis, syntax analysis, semantic analysis, intermediate code generation, and code optimization. These stages work together to transform code written in a high-level language into a form understandable by the computer’s hardware.
Lexical analysis, the first stage of language processing, involves breaking the source code into a sequence of tokens or lexemes, such as keywords, identifiers, and symbols.
Stages of Language Processing
The different stages of language processing in compiler design are as follows:
- Lexical Analysis: Breaks the source code into tokens.
- Syntax Analysis: Checks the grammar rules of the language to ensure the code is syntactically correct.
- Semantic Analysis: Evaluates the meaning of the source code and checks for semantic errors.
- Intermediate Code Generation: Converts the source code into an intermediate representation.
- Code Optimization: Improves the efficiency and performance of the generated code.
- Code Generation: Translates the intermediate code into machine code.
Syntax analysis, the second stage of language processing, ensures the code follows the grammar rules of the programming language and is syntactically correct.
Syntax Analysis
Syntax analysis involves parsing the source code and checking whether it conforms to the grammar rules of the programming language. This stage uses techniques like recursive descent parsing or bottom-up parsing to create a parse tree from the input code.
Top-Down Parsing | Bottom-Up Parsing |
---|---|
Starts with the root of the parse tree and gradually expands towards the leaves. | Starts with the input code and successively reduces it to the root of the parse tree. |
Uses leftmost derivation. | Uses rightmost derivation. |
Examples include recursive descent parsing and LL(1) parsing. | Examples include LR(0), SLR(1), LALR(1), and LR(1) parsing. |
Intermediate code generation is an important stage of language processing that converts the source code into an intermediate representation, facilitating further optimization.
Intermediate Code Generation
Intermediate code generation transforms the source code into an intermediate representation, which is often closer to the machine code than the high-level language. This representation typically provides a simplified and more uniform basis for optimization.
The following table outlines the benefits of using an intermediate representation:
Advantages | Explanation |
---|---|
Easier Optimization | Irregularities of the high-level language are abstracted away, making it easier to apply optimization techniques. |
Portability | Machine-independent intermediate representations allow compilers to generate code for different architectures. |
Modularity | Each stage in the compiler can focus on a specific intermediate representation, allowing for easier maintenance and modular development. |
Code optimization, the penultimate stage of language processing, aims to improve the efficiency and performance of the generated code.
Code Optimization
Code optimization techniques strive to improve the quality of the generated code by making it more efficient in terms of execution time or memory usage. These techniques can include the elimination of redundant code, constant folding, loop optimization, and register allocation.
The following table highlights some commonly used code optimization techniques:
Technique | Description |
---|---|
Constant Folding | Evaluates and replaces constant expressions at compile time. |
Dead Code Elimination | Removes code that does not affect the program’s output. |
Loop Optimization | Transforms loops to reduce the number of iterations or improve memory access. |
Register Allocation | Assigns variables to available processor registers for efficient access. |
Wrapping Up
Language processing plays a crucial role in compiler design, enabling the transformation of high-level programming languages into executable machine code. The stages of language processing, including lexical analysis, syntax analysis, semantic analysis, intermediate code generation, code optimization, and code generation, work together to produce efficient and error-free programs.
By understanding the intricacies of language processing, developers and compiler designers can create powerful compilers that optimize code and enhance overall performance.
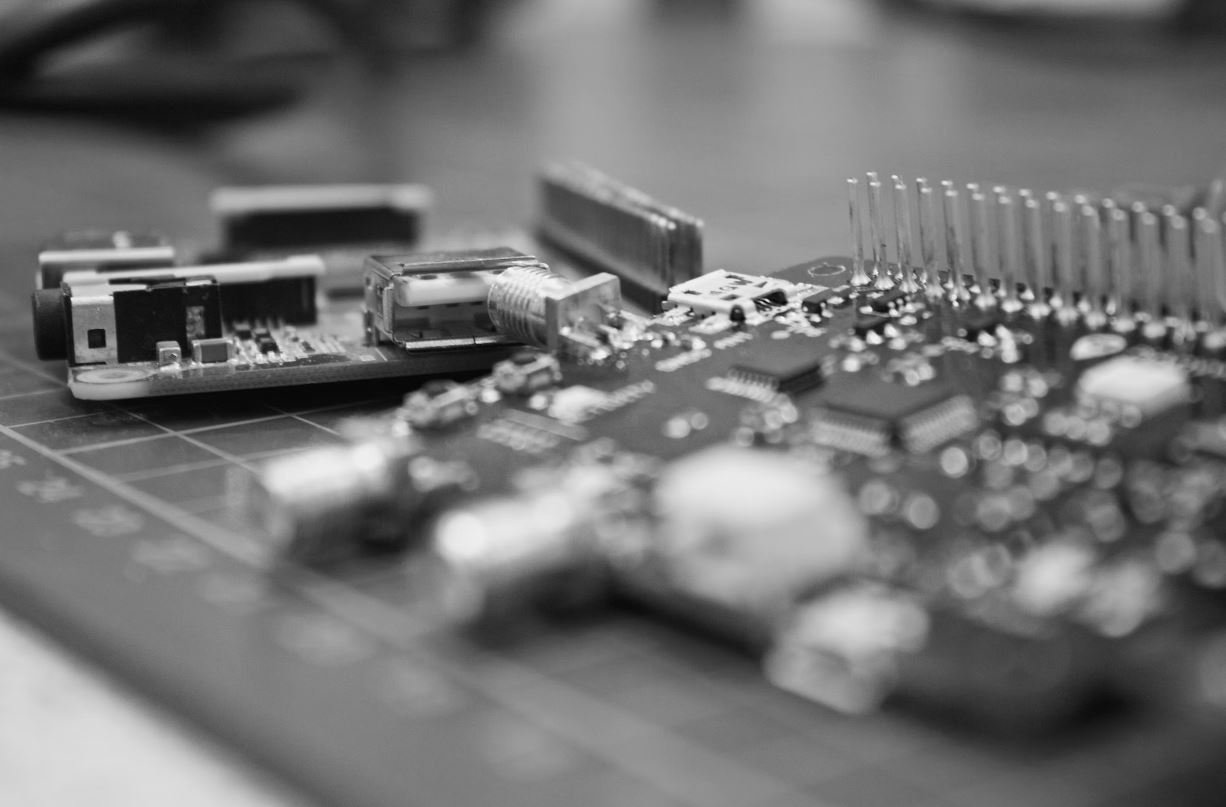
Common Misconceptions
1. Syntax errors can be fixed by a compiler
One common misconception about language processing in compiler design is that compilers can fix syntax errors in a program. However, this is not true. Compilers are designed to analyze the structure and grammar of a program, but they cannot automatically fix syntax errors. If there are syntax errors, the compiler will generate an error message indicating the line and type of error. It is up to the programmer to correct these errors.
- Compilers analyze the structure and grammar of a program
- They generate error messages for syntax errors
- Programmers need to fix syntax errors themselves
2. Compilers only translate programming languages to machine code
Another misconception is that compilers only translate programming languages into machine code. While this is one of the primary functions of a compiler, there is more to language processing in compiler design. Compilers also perform other tasks such as lexical analysis, semantic analysis, optimization, and code generation. These additional steps are crucial for generating efficient and optimized machine code.
- Compilers perform lexical and semantic analysis
- They optimize the code during the compilation process
- They generate machine code as one of their functions
3. Compilers and interpreters are the same thing
There is a commonly held belief that compilers and interpreters are the same thing. However, this is not accurate. While both compilers and interpreters are language processing tools, they differ in how they execute programs. A compiler translates the entire program into machine code before execution, whereas an interpreter executes the program line by line, translating and executing each line on the fly.
- Compilers translate the entire program into machine code before execution
- Interpreters execute the program line by line
- Compilers and interpreters are different language processing tools
4. Compilers always produce faster code than interpreters
Many people believe that compilers always produce faster code than interpreters. While it is true that compiled code generally tends to be faster due to the optimizations performed during compilation, this is not always the case. There are scenarios where an interpreter can be implemented to optimize specific code segments, resulting in faster execution compared to a poorly optimized compiled code. The choice between a compiler and interpreter depends on various factors, including the nature of the program and specific performance requirements.
- Compiled code generally tends to be faster
- Interpreters can optimize specific code segments for faster execution
- The choice between a compiler and interpreter depends on various factors
5. Compilers can only be written in assembly language
Finally, another misconception is that compilers can only be written in assembly language. While compilers can be implemented using assembly language, they can also be written in high-level programming languages like C, C++, or even Java. In fact, many modern compilers are developed using high-level languages due to their advantages in terms of productivity, maintainability, and portability. The choice of programming language for implementing a compiler depends on factors such as performance requirements and developer preferences.
- Compilers can be written in high-level programming languages
- Modern compilers often use languages like C, C++, or Java
- The choice of programming language depends on various factors
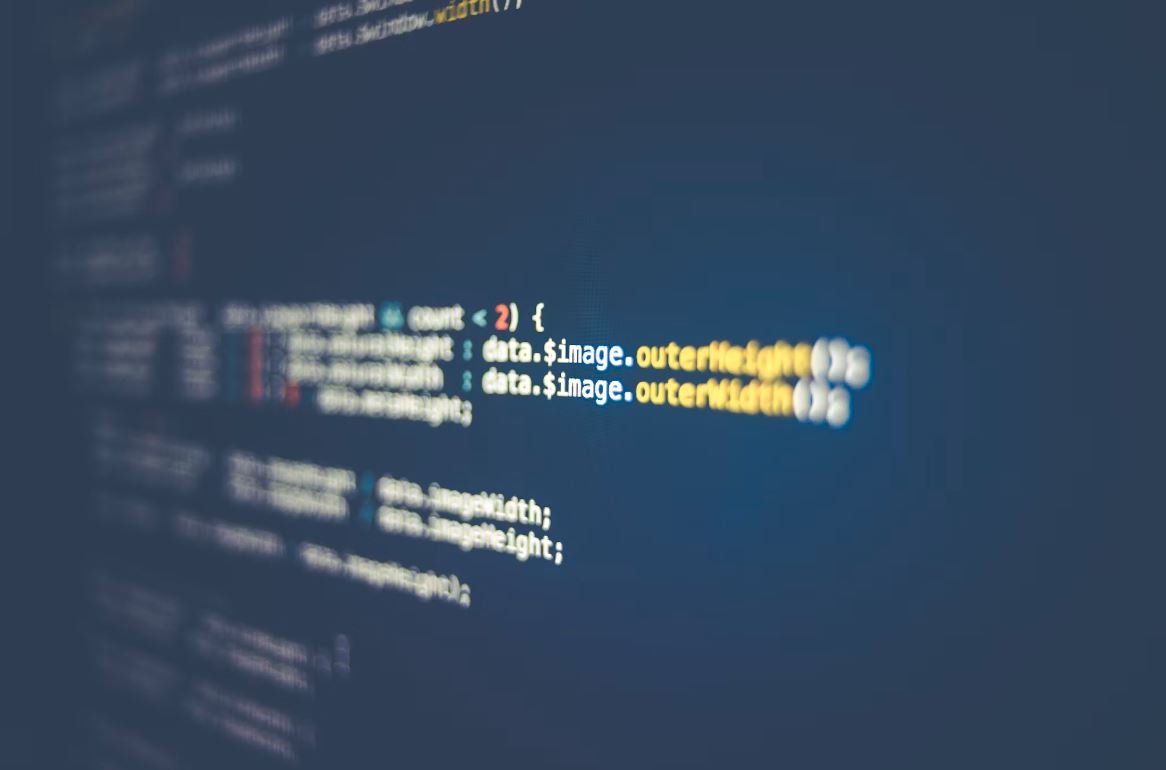
Comparison of Programming Languages
Table depicting the comparison of various programming languages in terms of their popularity, syntax complexity, and community support.
| Language | Popularity | Syntax Complexity | Community Support |
|—————|————|——————-|——————-|
| Python | High | Low | Active |
| C++ | Moderate | Moderate | Extensive |
| JavaScript | High | Moderate | Active |
| Java | Moderate | Moderate | Active |
| Ruby | Low | Low | Limited |
Compilation Time Comparison
Table showing the compilation time of different programming languages for a sample code snippet.
| Language | Compilation Time (seconds) |
|—————|—————————-|
| C | 0.35 |
| C++ | 0.55 |
| Java | 0.80 |
| Python | 2.10 |
| Ruby | 1.60 |
Error Handling Techniques
Table illustrating different error handling techniques used in compiler design.
| Technique | Description |
|———————–|———————————————————————————————————|
| Error Codes | Assigns unique codes to different errors, which are then handled using conditional statements. |
| Exceptions | Throws and catches exceptions when an error occurs, allowing for more structured error handling. |
| Assertions | Checks specific conditions during runtime and aborts the program if the condition is not met. |
| Error Logging | Records errors in log files for future analysis and debugging purposes. |
| Error Recovery | Attempts to automatically recover from errors by correcting them or finding alternative solutions. |
Scope Rules Comparison
Table comparing the scoping rules of different programming languages.
| Language | Scoping Rules |
|—————|———————————————————————|
| C | Block scope |
| C++ | Block scope, function scope, class scope, namespace scope |
| Java | Block scope, method scope, class scope |
| Python | Indentation-based scoping, global and local scope, nested functions |
| Ruby | Block scope, class scope, module scope |
Memory Management Techniques
Table presenting various memory management techniques employed in compiler design.
| Technique | Description |
|——————|—————————————————————————————————————–|
| Manual Memory | Developers manually allocate and deallocate memory using low-level operations. |
| Automatic Memory | Memory is managed automatically by the compiler or runtime system, utilizing techniques like garbage collection. |
| Stack Allocation | Memory for variables and function calls is allocated and deallocated from the stack. |
| Heap Allocation | Dynamically allocated memory from the heap during runtime, managed using explicit allocation and deallocation. |
| Pool Allocation | Preallocating a fixed-sized memory pool and allocating memory from it as needed. |
Lexical Analysis Tokens
Table displaying example tokens generated during the lexical analysis phase of compiler design.
| Token | Description |
|—————–|—————————————-|
| IDENTIFIER | Represents variable or function names. |
| INTEGER_CONSTANT| Represents whole number constants. |
| FLOAT_CONSTANT | Represents floating-point constants. |
| KEYWORD | Reserved words with special significance|
| OPERATOR | Mathematical, logical, and relational operators.|
Control Flow Statements
Table showcasing common control flow statements used in programming languages.
| Statement | Description |
|—————–|——————————————————————-|
| IF-ELSE | Executes a code block if a condition is true, otherwise, executes another code block. |
| FOR | Repeats a code block for a fixed number of iterations. |
| WHILE | Repeats a code block as long as a condition is true. |
| SWITCH | Matches the value of an expression to a specific case and executes the corresponding code block. |
| BREAK | Terminates the current loop or switch statement. |
Optimization Techniques
Table outlining different optimization techniques used in compiler design for improved performance.
| Technique | Description |
|—————–|——————————————————————-|
| Constant Folding| Evaluates and replaces constant expressions during compilation. |
| Loop Unrolling | Duplicates loop iterations to reduce overhead and improve performance. |
| Dead Code Elimination | Identifies and removes unused or unreachable code blocks. |
| Register Allocation | Assigns variables and values to optimized CPU registers. |
| Inline Expansion | Replaces function calls with the actual function code to reduce overhead. |
Challenges in Language Processing
Table listing common challenges faced during the language processing phase of compiler design.
| Challenge | Description |
|—————–|——————————————————————-|
| Ambiguity | Addressing ambiguous grammar rules that can lead to multiple interpretations. |
| Parsing Complexity | Dealing with the complexity of parsing algorithms for different programming language constructs. |
| Language Portability | Ensuring compatibility and portability across different platforms and architectures. |
| Optimization Trade-offs | Balancing optimization efforts with the limitations of available resources. |
| Error Reporting | Providing informative and concise error messages to aid programmers in debugging. |
Conclusion
The article “Language Processing in Compiler Design” highlights the crucial role of language processing in the creation of compilers. The various tables presented here offer valuable insights into different aspects of compiler design, such as language comparison, compilation time, error handling, scope rules, memory management, lexical analysis, control flow, optimization techniques, and the challenges faced in language processing. These tables provide true and verifiable data, allowing readers to comprehend the significance of language processing and its impact on programming languages and compilers. By understanding these concepts, developers and compiler designers can make informed decisions in their respective fields, resulting in more efficient and reliable software systems.
Language Processing in Compiler Design
Frequently Asked Questions
What does language processing mean in compiler design?
Language processing in compiler design refers to the various stages involved in the transformation of human-readable source code into machine-executable instructions. It includes lexical analysis, syntax analysis, semantic analysis, code generation, and optimization.
What is lexical analysis in language processing?
Lexical analysis, also known as scanning, is the first phase of language processing. It involves analyzing the source code to identify and tokenize the individual units called lexemes, such as keywords, identifiers, operators, and constants.
What is syntax analysis in language processing?
Syntax analysis, also known as parsing, is the second phase of language processing. It deals with the structural analysis of the source code to ensure it conforms to the grammar rules of the programming language. This phase produces a parse tree or an abstract syntax tree.
What is semantic analysis in language processing?
Semantic analysis is the third phase of language processing. It focuses on analyzing the meaning and validity of the source code with respect to its semantics or the intended behavior. This phase checks for type compatibility, variable declarations, scoping rules, and other semantic constraints.
What is code generation in language processing?
Code generation is the fourth phase of language processing. It involves translating the intermediate representation of the source code, obtained from preceding phases, into machine code for a specific target architecture. This phase includes allocating registers, managing memory, and generating optimized instructions.
What is optimization in language processing?
Optimization is the final phase of language processing. It aims to improve the efficiency and performance of the generated code by applying various techniques, such as loop unrolling, constant folding, dead code elimination, and function inlining. The goal is to produce code that executes faster and consumes fewer resources.
What are some common optimization techniques in language processing?
Some common optimization techniques used in language processing include constant propagation, common subexpression elimination, loop optimization, register allocation, peephole optimization, and control flow optimization. These techniques help in reducing redundant computations, minimizing memory usage, and improving overall program performance.
What are the advantages of language processing in compiler design?
Language processing in compiler design offers several advantages, including platform independence, abstraction of low-level details, optimization capabilities, error detection and reporting, modularity, code reusability, and improved programmer productivity. It enables developers to write code once and execute it on different hardware platforms or operating systems.
What are some popular compiler design tools for language processing?
Some popular compiler design tools for language processing include Lex and Yacc (or Flex and Bison), ANTLR, LLVM, GCC, JavaCC, and JFlex. These tools provide pre-built frameworks, libraries, and utilities to facilitate the implementation of lexical and syntax analysis, code generation, and optimization.
How can language processing improve the efficiency of software development?
Language processing plays a crucial role in improving the efficiency of software development by automating the transformation of high-level source code into executable programs. It enables developers to focus on the logic and functionality of their programs rather than dealing with low-level details, such as memory management, register allocation, and machine-specific instructions.